Playing with the Spotify Connect API

José M. Pérez / April 22, 2017
6 min read • 5,113 views
Spotify released recently a set of endpoints in beta to fetch information of what is playing and send playback commands. This allows for a wide range of integrations and I wanted to hack a bit with it.
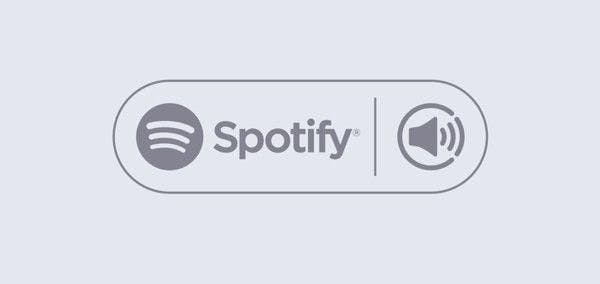
What is Spotify Connect
Spotify Connect is a way to transmit the playback from one device to another one without having to use a physical connection like a cable or bluetooth. You can send the music from your Spotify desktop client to a speaker, from the Spotify app on mobile to Spotify for PlayStation, from the Spotify web player to Chromecast… In short, you have controllers and devices that can play music.
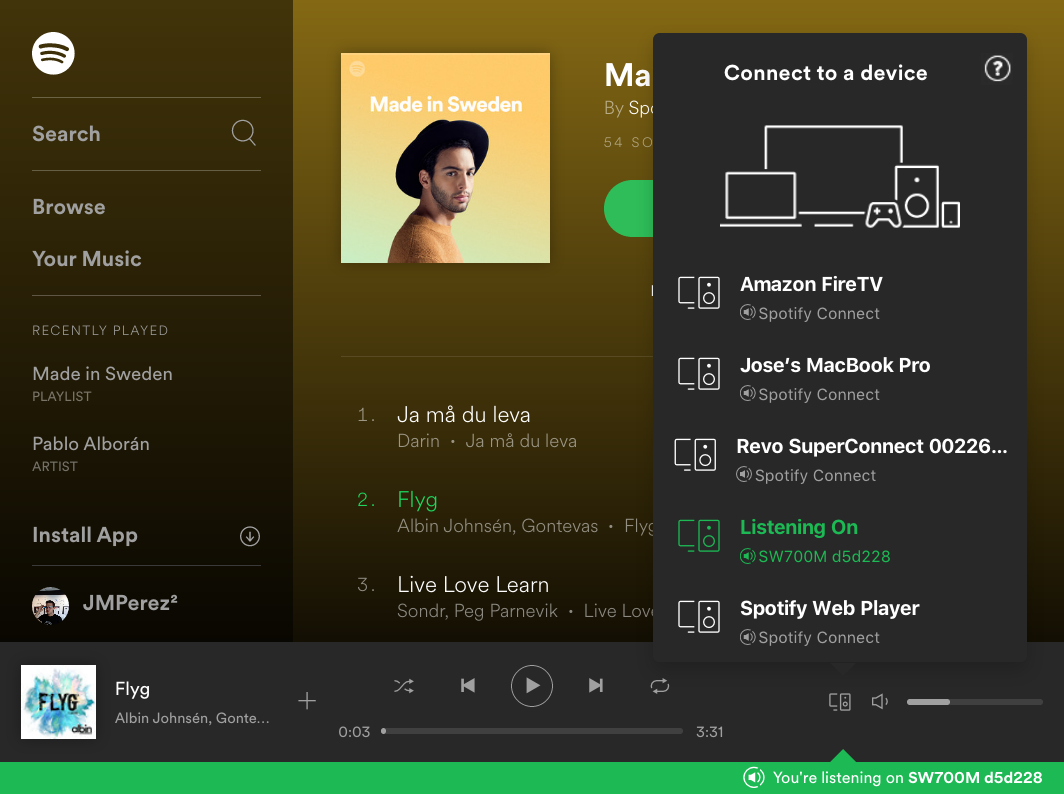
Spotify's Web Player (zoomed in). Clicking on the icon next to the volume we get a list of connected devices (FireTV, desktop client, speakers and the web player).
Your application can become a controller through the Web API endpoints, getting information about what is playing currently and from where, being able to transmit the playback to another connected device or interact with the current context (pausing, changing the volume, skipping, playing something else…).
How to use the endpoints
Before using the Connect endpoints we need to obtain an access token on behalf of the user with certain permissions. There is more information about what scopes are needed in the documentation for each endpoint.
You don't need to have a premium account to get the playback status, a free account is alright. You will do need a premium one if you want to send commands to change the playback.
A caveat at the moment is that the endpoints don't support any kind of web socket connection nor long polling. Thus, if you want to get updates on the position of the current playing track or any other change in the context, you need to poll every few seconds.
A small library to make it easier to use the endpoints
The trickiest part of using the Spotify Web API is to implement the authorization flow. The Authorization Guide does a good job explaining it, but I thought I could do something so developers wouldn't need to worry about setting up the whole flow, hiding away the authentication and just getting.
That's why I have created spotify-player. It's both a server and a library that you use to communicate with it. To use it, you just need to include a script, call login() and subscribe to the updates:
<script src="https://spotify-player.herokuapp.com/spotify-player.js"></script>
var spotifyPlayer = new SpotifyPlayer();
spotifyPlayer.on('update', response => {
// render the track received
});
spotifyPlayer.on('login', user => {
if (user === null) {
// no user
} else {
// say hello to the user, and tell them to play something!
}
});
spotifyPlayer.init();
spotifyPlayer.login();
You can forget about setting up a Spotify application and a server, carrying out the token exchange, token refresh, and persisting the current user, so you can focus on the fun part.
Other methods include a function to make calls to other Spotify endpoints reusing the same access token, so you can fetch other data that can help you creating a more complete visualisation.
Let's have a look at this pen as an example of a basic visualisation:
And in case you can't try it or don't have a Spotify account this is pretty much what it looks like:
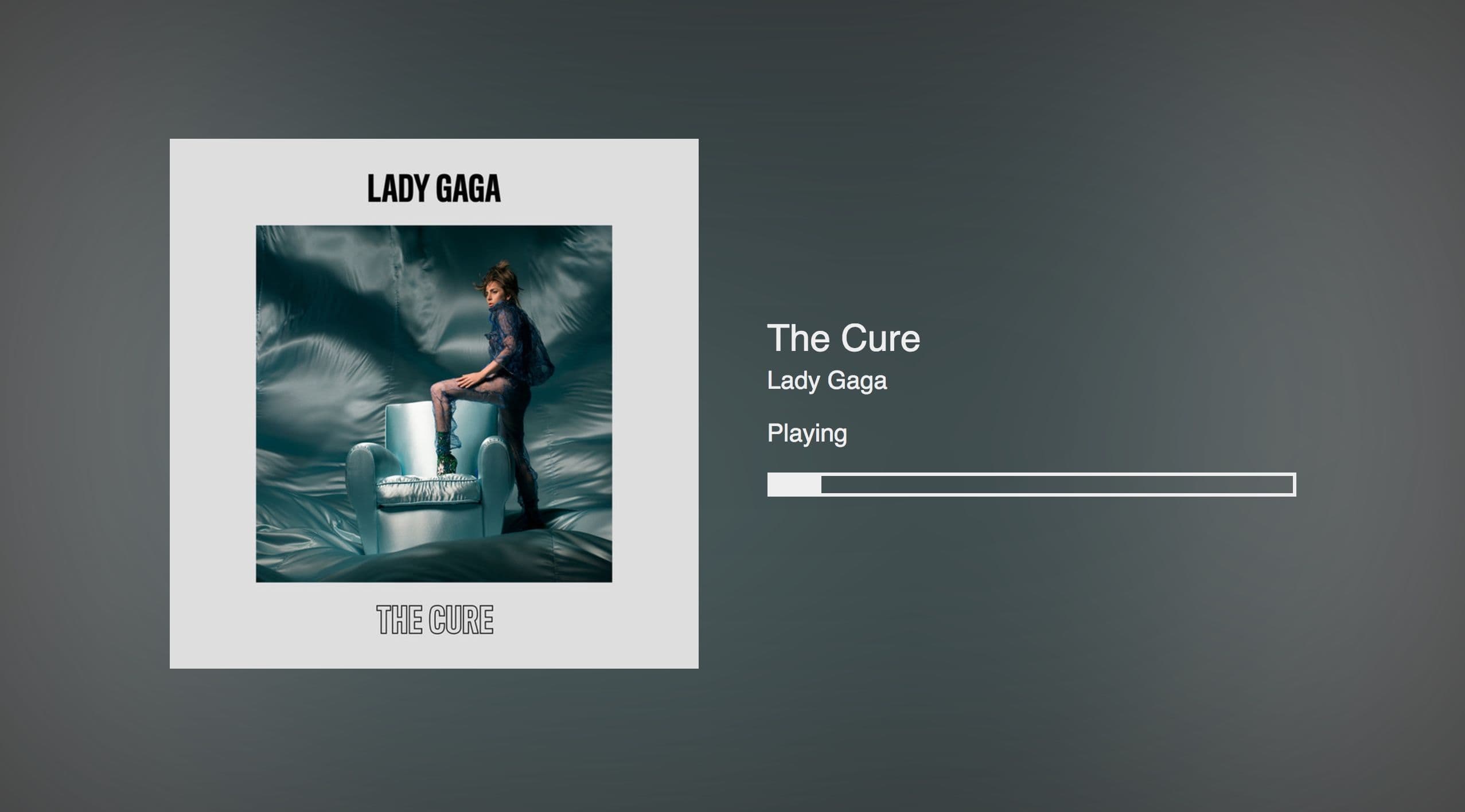
I have kept the example very basic since the point is understanding the usage of the library. If you are into performance and UX you'll see there is room for improvement, but as a front-end developer I know an example can get out of hand very easily when adding things.
I encourage you to fork it and start making your own visualisation. And once you do it, ping me so I include it in this Codepen collection.
Possible applications
Disclaimer: This is a list of some use cases. You still need to comply with Spotify's terms of use when implementing an application that uses Spotify'sWeb API. This might mean adding certain messaging and link to the song in Spotify.
Dynamic visualisations
You could combine the playback position with the audio analysis of the track to generate dynamic visualisations using loudness, tempo, key, timbre or pitch of the segments that compose the track. You can also use the endpoint to fetch audio features of a track, which gives you high level information about characteristics of the song.
See the Pen on Codepen and Possan's original visualisation on GitHub
Now playing view
Are you a coffee-shop owner and people always wonder what song is playing? You could have a TV showing a branded now playing view. You could even have a widget on your website, or a script posting to a social network what is currently playing so your customers know. Or a small browser extension showing what is playing and/or showing desktop notifications when the track changes.
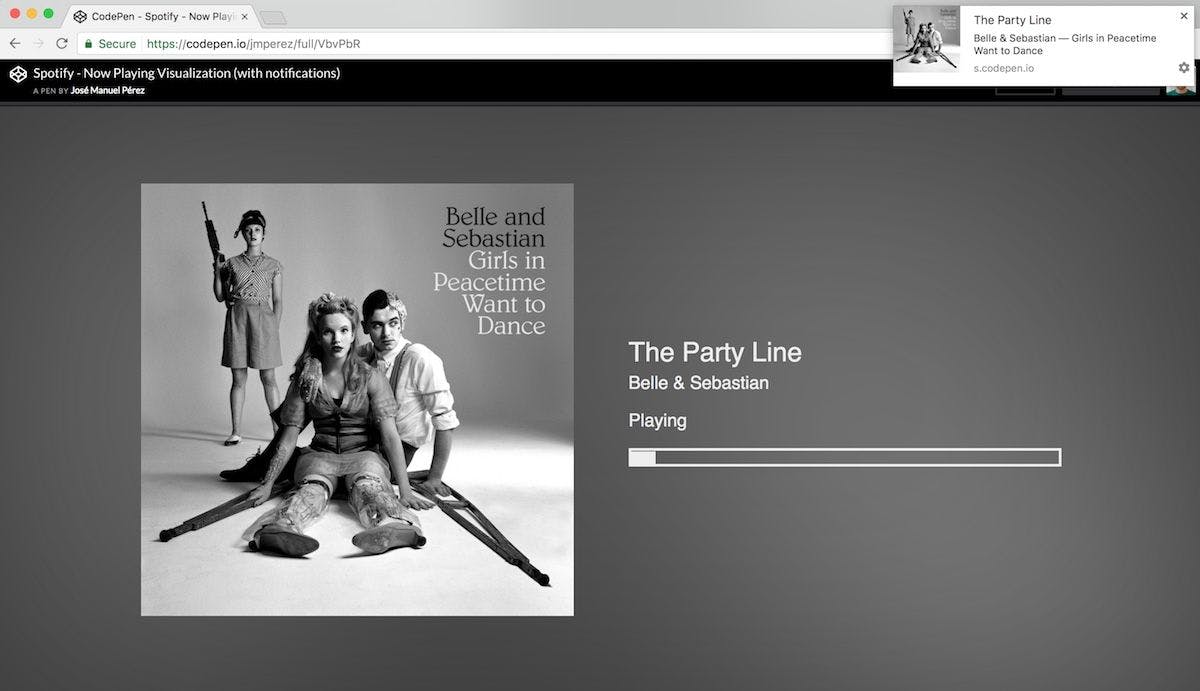
See the Pen on Codepen. When the track changes the browser shows a Web Notification.
Or if you are at home hosting a dinner or party, show on the TV or computer what is playing.
You can combine other Spotify Web API endpoints too. Eg you could fetch the artists info to show the artist profile image in the background:
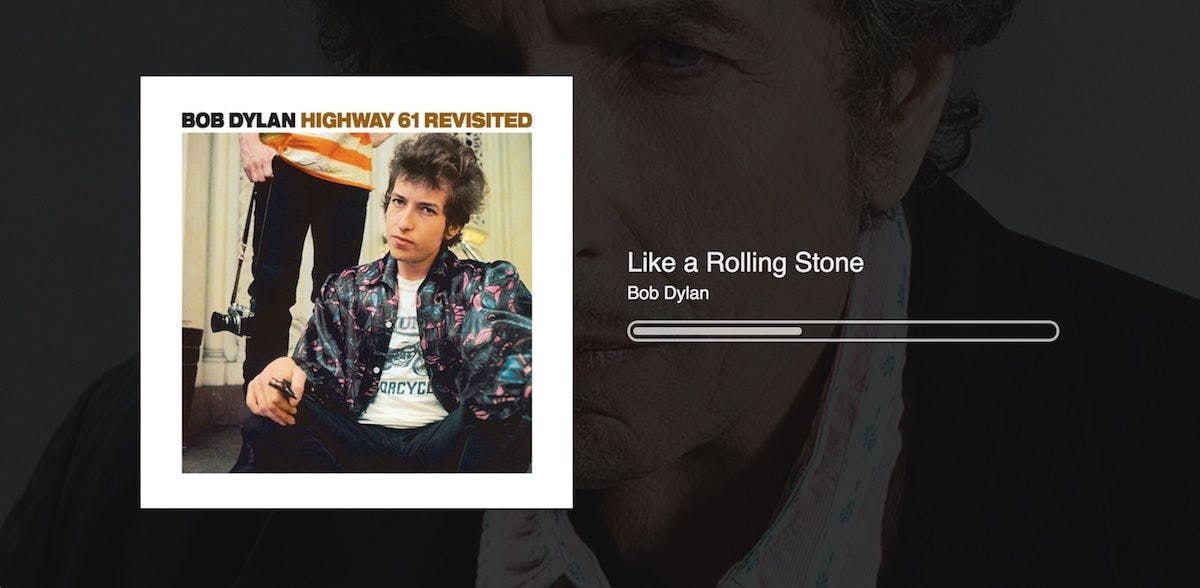
See the Pen on Codepen. A simple visualisation of what's playing in Spotify rendering the artist profile image as the background.
You could use an API like Musixmatch's and create an app or a website showing the lyrics for the current song, synchronised with the playback position.
And your imagination is the limit. Use Genius' API to get annotations about a song, or search for trivia and more info about the song or artist using Wikipedia's API. And if you don't want to miss what's happening in the world, implement a news ticker in your view using News API.
MVP
The library is really small and it only supports reading the playback state and not send commands. Let's call it an MVP. I might work on adding more features in the future.